New JavaScript Set methods
Explore the latest JavaScript Set methods that enhance functionality and improve efficiency in handling collections. These new methods simplify operations like intersection, union, and difference, making it easier to manage unique data. Perfect for developers looking to optimize their code with powerful and intuitive tools.
New JavaScript Set Methods: A Comprehensive Guide
In the dynamic world of JavaScript, the Set
object is a powerful feature that allows you to store unique values of any type. JavaScript's Set
methods have evolved, offering new functionalities that can enhance the efficiency and capabilities of your code. This article delves into the latest Set
methods, explaining their uses and how they can improve your coding practices.
Understanding the JavaScript Set Object
The JavaScript Set
object is a collection of values where each value must be unique. This makes it an excellent choice for scenarios where you need to ensure no duplicate values are stored. Unlike arrays, Set
objects do not allow duplicate values and maintain insertion order. They provide several methods to manipulate and access the stored values efficiently.
New Methods in JavaScript Sets
Recent updates to JavaScript have introduced several new methods to the Set
object, expanding its functionality and providing developers with more tools to work with. Here’s a closer look at these new methods:
Set.prototype.union
The union
method allows you to combine two sets into one. This method creates a new Set
containing all unique values from both sets. It’s useful for merging sets without worrying about duplicates.
Usage Example:
const set2 = new Set([3, 4, 5]);
const unionSet = set1.union(set2);
console.log([...unionSet]); // Output: [1, 2, 3, 4, 5]
const set1 = new Set([1, 2, 3]); const set2 = new Set([3, 4, 5]); const unionSet = set1.union(set2); console.log([...unionSet]); // Output: [1, 2, 3, 4, 5]
Set.prototype.intersection
The intersection
method returns a new Set
that contains only the values present in both sets. This method is particularly helpful when you need to find common elements between two sets.
Usage Example:
const set2 = new Set([2, 3, 4]);
const intersectionSet = set1.intersection(set2);
console.log([...intersectionSet]); // Output: [2, 3]
const set1 = new Set([1, 2, 3]); const set2 = new Set([2, 3, 4]); const intersectionSet = set1.intersection(set2); console.log([...intersectionSet]); // Output: [2, 3]
Set.prototype.difference
The difference
method provides a new Set
containing values that are present in the first set but not in the second. This method helps you identify unique elements in one set compared to another.
Usage Example:
const set2 = new Set([2, 3, 4]);
const differenceSet = set1.difference(set2);
console.log([...differenceSet]); // Output: [1]
const set1 = new Set([1, 2, 3]); const set2 = new Set([2, 3, 4]); const differenceSet = set1.difference(set2); console.log([...differenceSet]); // Output: [1]
Set.prototype.symmetricDifference
The symmetricDifference
method returns a new Set
with values that are unique to either of the sets, but not in both. This method is useful for finding values that are present in one set or the other, but not in both.
Usage Example:
const set2 = new Set([2, 3, 4]);
const symmetricDifferenceSet = set1.symmetricDifference(set2);
console.log([...symmetricDifferenceSet]); // Output: [1, 4]
const set1 = new Set([1, 2, 3]); const set2 = new Set([2, 3, 4]); const symmetricDifferenceSet = set1.symmetricDifference(set2); console.log([...symmetricDifferenceSet]); // Output: [1, 4]
Set.prototype.isSubsetOf
The isSubsetOf
method checks whether all elements of one set are also present in another set. This is particularly useful for validating subset relationships.
Usage Example:
const set2 = new Set([1, 2, 3]);
const isSubset = set1.isSubsetOf(set2);
console.log(isSubset); // Output: true
const set1 = new Set([1, 2]); const set2 = new Set([1, 2, 3]); const isSubset = set1.isSubsetOf(set2); console.log(isSubset); // Output: true
Set.prototype.isSupersetOf
The isSupersetOf
method determines if a set contains all elements of another set. This method is useful for checking if one set is a superset of another.
Usage Example:
const set2 = new Set([1, 2]);
const isSuperset = set1.isSupersetOf(set2);
console.log(isSuperset); // Output: true
const set1 = new Set([1, 2, 3]); const set2 = new Set([1, 2]); const isSuperset = set1.isSupersetOf(set2); console.log(isSuperset); // Output: true
Set.prototype.clearAll
The clearAll
method allows you to remove all elements from a set in one operation. This method is particularly handy for clearing the entire set efficiently.
Usage Example:
set.clearAll();
console.log(set.size); // Output: 0
const set = new Set([1, 2, 3]); set.clearAll(); console.log(set.size); // Output: 0
Implementing New Set Methods
To use these new methods, ensure your JavaScript environment supports them. They are available in modern JavaScript environments but might require polyfills or transpilation in older environments.
Best Practices for Using JavaScript Sets
- Leverage Unique Value Property: Utilize the uniqueness property of
Set
objects to manage collections of unique items effectively. - Combine with Other Data Structures: Use
Set
in combination with arrays, maps, and other data structures to enhance functionality and achieve complex results. - Optimize Performance: Consider the performance implications of using
Set
methods, especially with large data sets. Use the most efficient methods for your specific needs.
FAQs
Q1: Are these new Set
methods supported in all JavaScript environments?
A1: The new Set
methods might not be supported in all environments. Ensure compatibility with your target environment or use polyfills if necessary.
Q2: How can I use these methods in older JavaScript environments?
A2: For older environments, consider using polyfills or transpilers like Babel to ensure compatibility. Check the documentation for specific polyfill libraries that support these methods.
Q3: Can I use these methods on Set
objects created from arrays?
A3: Yes, these methods can be used on Set
objects regardless of how they were created. They operate on the unique values contained in the Set
.
Q4: Are there performance considerations when using these methods?
A4: Performance can vary based on the size of the sets and the complexity of the operations. For large sets, consider profiling your code to ensure it meets performance requirements.
Q5: How do these methods compare to similar array methods?
A5: While similar operations can be performed on arrays, Set
methods provide more efficient ways to handle unique values and set operations, avoiding the need for manual deduplication and complex logic.
By utilizing these new JavaScript Set
methods, you can streamline your code and perform set operations more effectively. Embrace these enhancements to boost your coding efficiency and maintainability.
Get in Touch
Website – https://www.webinfomatrix.com
Mobile - +91 9212306116
Whatsapp – https://call.whatsapp.com/voice/9rqVJyqSNMhpdFkKPZGYKj
Skype – shalabh.mishra
Telegram – shalabhmishra
Email - info@webinfomatrix.com
What's Your Reaction?
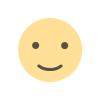
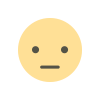
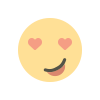
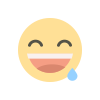
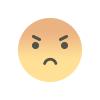
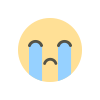
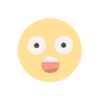